Calendar
Mo | Tu | We | Th | Fr | Sa | Su |
---|
30 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | 29 | 30 | 31 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
Archive
- 2002
- 2003
- 2004
- 2005
- 2006
- 2007
- 2008
- 2009
- 2010
- 2011
- 2012
- 2013
- 2014
- 2015
- 2016
- 2019
- 2020
|
Website may be up and down over next few months. I'm currently doing a complete overhaul of everything.
Going back to simple individual .htm pages, new overall site theme, sanitizing and cleaning up html of all
pages and blog posts, attempting to implement a new tooling and publishing system etc etc.
/// <summary>Gets the value associated with the specified key and casts the value to the desired type.</summary>
/// <returns>true if the <see cref="T:System.Collections.Generic.Dictionary`2" /> contains an element with the specified key; otherwise, false.</returns>
/// <param name="dictionary">The dictionary to retrieve the value from.</param>
/// <param name="key">The key of the value to get.</param>
/// <param name="value">When this method returns, contains the value associated with the specified key, if the key is found; otherwise, the default value for the type of the <paramref name="value" /> parameter. This parameter is passed uninitialized.</param>
public static bool TryGetValueCast<T, V, C>(this IDictionary<T, V> dictionary, T name, out C value)
{
return TryGetValueCast(dictionary, name, out value, default(C));
}
/// <summary>Gets the value associated with the specified key and casts the value to the desired type.</summary>
/// <returns>true if the <see cref="T:System.Collections.Generic.Dictionary`2" /> contains an element with the specified key; otherwise, false.</returns>
/// <param name="dictionary">The dictionary to retrieve the value from.</param>
/// <param name="key">The key of the value to get.</param>
/// <param name="value">When this method returns, contains the value associated with the specified key, if the key is found; otherwise, the default value for the type of the <paramref name="value" /> parameter. This parameter is passed uninitialized.</param>
/// <param name="defaultValue">The default value to return if unable to fetch using key.</param>
public static bool TryGetValueCast<T, V, C>(this IDictionary<T, V> dictionary, T key, out C value, C defaultValue)
{
if (dictionary == null)
{
throw new ArgumentNullException("dictionary");
}
try
{
value = (C)Convert.ChangeType(dictionary[key], typeof(C));
}
catch
{
value = defaultValue;
return false;
}
return true;
}
e7c04853-9d7e-401a-8c66-abe7d4656c5f|0|.0
The code snippet below allows you to attach a translation gizmo to a object to allow the user to move that object while the game is running. Works just like the unity translation gizmo. 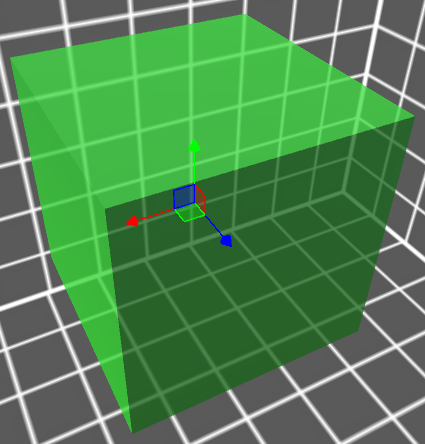 using System;
using UnityEngine;
/// <summary>
/// The translation gizmo.
/// </summary>
public class TranslationGizmo : MonoBehaviour
{
#region Fields
/// <summary>
/// The camera reference used to determin distance to translation gizmo.
/// </summary>
public UnityEngine.Camera cameraReference;
/// <summary>
/// Used to determine how thick the envelope is around a axis line when attempting to click and drag it with the mouse.
/// </summary>
public float axisEnvelope = 0.04f;
/// <summary>
/// Determines the length of the cone.
/// </summary>
public float coneLength = 0.85f;
/// <summary>
/// Specifies how many segments the cone is made up of.
/// </summary>
public int coneSegments = 30;
/// <summary>
/// Determines the diameter of the cone.
/// </summary>
public float coneSize = 0.075f;
/// <summary>
/// Determines the length of the axis lines.
/// </summary>
public float lineLength = 0.13f;
/// <summary>
/// The line material used to render the lines.
/// </summary>
public Material lineMaterial;
/// <summary>
/// Determines the size of the square panning gizmos.
/// </summary>
public float panScale = 0.3f;
/// <summary>
/// Determines the transparency level of the axis plane quads.
/// </summary>
public float planeTransparency = 0.3f;
/// <summary>
/// The color that will be used for the X axis.
/// </summary>
public Color xAxisColor = Color.red;
/// <summary>
/// The color that will be used for the Y axis.
/// </summary>
public Color yAxisColor = Color.green;
/// <summary>
/// The color that will be used for the Z axis.
/// </summary>
public Color zAxisColor = Color.blue;
/// <summary>
/// Holds the button state used to determine if dragging has started.
/// </summary>
protected bool inputButtonState;
/// <summary>
/// Holds the relative mouse X position.
/// </summary>
protected float mouseAxisX;
/// <summary>
/// Holds the relative mouse Y position.
/// </summary>
protected float mouseAxisY;
/// <summary>
/// Holds the pre-calculated distance value from the camera to the game object.
/// </summary>
private float cameraDistance;
/// <summary>
/// The hit mouse down.
/// </summary>
private Vector3 hitMouseDown;
/// <summary>
/// The hit mouse move.
/// </summary>
private Vector3 hitMouseMove;
/// <summary>
/// Holds wheather or not the input axis has changed.
/// </summary>
private bool inputAxisChanged;
/// <summary>
/// Holds wheather or not the mouse if being dragged.
/// </summary>
private bool isDragging;
/// <summary>
/// Holds XY plane information.
/// </summary>
private Plane planeXY;
/// <summary>
/// Holds XZ plane information.
/// </summary>
private Plane planeXZ;
/// <summary>
/// Holds YZ plane information.
/// </summary>
private Plane planeYZ;
/// <summary>
/// Holds the previous input button state.
/// </summary>
private bool previousInputButtonState;
/// <summary>
/// Holds the rotation matrix used to determine at what rotation the gizmo will be drawn at.
/// </summary>
private Matrix4x4 rotationMatrix;
/// <summary>
/// Holds the value for the <see cref="WorldTranslation"/> property.
/// </summary>
[SerializeField]
private bool worldTranslation;
/// <summary>
/// Gets or sets a value indicating whether world translation is enabled.
/// </summary>
/// <value>
/// <c>true</c> if world translation is active; otherwise, <c>false</c> for local translation.
/// </value>
public bool WorldTranslation
{
get
{
return this.worldTranslation;
}
set
{
this.worldTranslation = value;
}
}
/// <summary>
/// Holds the type of axis movement is currently being made.
/// </summary>
private TypeOfMove typeMovementBeingMade;
#endregion
#region Public Events
/// <summary>
/// Occurs when position changed.
/// </summary>
public event EventHandler<Vector3EventArgs> PositionChanged;
#endregion
#region Enums
/// <summary>
/// Enum defining the types of axis movement.
/// </summary>
private enum TypeOfMove
{
/// <summary>
/// No axis movement.
/// </summary>
None,
/// <summary>
/// Movement along the X axis.
/// </summary>
XAxis,
/// <summary>
/// Movement along the
/// </summary>
X2Axis,
/// <summary>
/// Movement along the Y axis.
/// </summary>
YAxis,
/// <summary>
/// The y 2 axis.
/// </summary>
Y2Axis,
/// <summary>
/// Movement along the Z axis.
/// </summary>
ZAxis,
/// <summary>
/// The z 2 axis.
/// </summary>
Z2Axis,
/// <summary>
/// Movement along the XZ axis.
/// </summary>
XZAxis,
/// <summary>
/// Movement along the YZ axis.
/// </summary>
YZAxis,
/// <summary>
/// Movement along the XY axis.
/// </summary>
XYAxis,
};
#endregion
#region Public Methods and Operators
/// <summary>
/// Awake is called when the script instance is being loaded.
/// </summary>
public void Awake()
{
// if no material assigned setup a default material
if (this.lineMaterial == null)
{
#if !UNITY_5
this.lineMaterial =
new Material(
"Shader \"Lines/Colored Blended\" {" + "SubShader { Pass { " + " Blend SrcAlpha OneMinusSrcAlpha "
+ " ZWrite Off Cull Off Fog { Mode Off } ZTest Always" + " BindChannels {"
+ " Bind \"vertex\", vertex Bind \"color\", color }" + "} } }");
#else
// Unity has a built-in shader that is useful for drawing
// simple colored things.
var shader = Shader.Find("Hidden/Internal-Colored");
this.lineMaterial = new Material(shader);
this.lineMaterial.hideFlags = HideFlags.HideAndDontSave;
// Turn on alpha blending
this.lineMaterial.SetInt("_SrcBlend", (int)UnityEngine.Rendering.BlendMode.SrcAlpha);
this.lineMaterial.SetInt("_DstBlend", (int)UnityEngine.Rendering.BlendMode.OneMinusSrcAlpha);
// Turn backface culling off
this.lineMaterial.SetInt("_Cull", (int)UnityEngine.Rendering.CullMode.Off);
// Turn off depth writes
this.lineMaterial.SetInt("_ZWrite", 0);
#endif
}
}
/// <summary>
/// Update is called every frame, if the MonoBehaviour is enabled.
/// </summary>
public void Update()
{
if (cameraReference == null)
{
return;
}
// calculate distance once between the camera and the game objects position
var transformReference = this.transform;
var position = transformReference.position;
this.cameraDistance = Vector3.Distance(this.cameraReference.transform.position, position) * this.lineLength;
this.previousInputButtonState = this.inputButtonState;
this.GetInputButtonState();
// if the input button is released stop moving
if (!this.inputButtonState)
{
this.isDragging = false;
this.typeMovementBeingMade = TypeOfMove.None;
return;
}
// only handle dragging is already dragging or if input button state was pressed
if (this.isDragging || (!this.previousInputButtonState && this.inputButtonState))
{
// get mouse/input values
this.GetInputValues();
this.inputAxisChanged = Math.Abs(this.mouseAxisX) > float.Epsilon || Math.Abs(this.mouseAxisY) > float.Epsilon;
// update planes based on world or local translation
if (this.worldTranslation)
{
// update planes
this.planeXZ.SetNormalAndPosition(Vector3.up, position);
this.planeXY.SetNormalAndPosition(Vector3.forward, position);
this.planeYZ.SetNormalAndPosition(Vector3.right, position);
}
else
{
// update planes
this.planeXZ.SetNormalAndPosition(transformReference.up, position);
this.planeXY.SetNormalAndPosition(transformReference.forward, position);
this.planeYZ.SetNormalAndPosition(transformReference.right, position);
}
// cast a ray once
var ray = this.cameraReference.ScreenPointToRay(Input.mousePosition);
// handle axis translations
this.HandleXZ(ray);
this.HandleXY(ray);
this.HandleYZ(ray);
}
}
#endregion
#region Methods
/// <summary>
/// Get the state of the input button used to determine if dragging has occurred.
/// </summary>
protected virtual void GetInputButtonState()
{
this.inputButtonState = Input.GetMouseButton(0);
}
/// <summary>
/// Gets the X/Y input values
/// </summary>
protected virtual void GetInputValues()
{
this.mouseAxisX = Input.GetAxis("Mouse X");
this.mouseAxisY = Input.GetAxis("Mouse Y");
}
/// <summary>
/// Raises the <see cref="PositionChanged"/> event.
/// </summary>
/// <param name="previousPosition">
/// The previous Position.
/// </param>
/// <param name="position">
/// The position.
/// </param>
protected virtual void OnPositionChanged(Vector3 previousPosition, Vector3 position)
{
var handler = this.PositionChanged;
if (handler != null)
{
handler(this, new Vector3EventArgs(previousPosition, position));
}
}
/// <summary>
/// Uses OpenGL to draw a line for the axis.
/// </summary>
/// <param name="color">
/// The color to use when drawing the cone.
/// </param>
/// <param name="axis">
/// Defines axis that the cone will be drawn against.
/// </param>
/// <param name="axisU">
/// Represents the "X" axis to draw the base of the cone against.
/// </param>
/// <param name="axisV">
/// Represents the "Y" axis to draw the base of the cone against.
/// </param>
private void DrawAxis(Color color, Vector3 axis, Vector3 axisU, Vector3 axisV)
{
// scale the axis by the camera distance
axis = axis * this.cameraDistance;
// start drawing lines
GL.Begin(GL.LINES);
GL.Color(color);
GL.Vertex3(0, 0, 0);
GL.Vertex3(axis.x, axis.y, axis.z);
GL.End();
// draw the cone for the axis
this.DrawCone(axis, axisU, axisV, this.cameraDistance * this.coneSize, this.coneLength, color);
}
/// <summary>
/// Uses OpenGL to draw a cone for the axis.
/// </summary>
/// <param name="axis">
/// Defines axis that the cone will be drawn against.
/// </param>
/// <param name="axisU">
/// Represents the "X" axis to draw the base of the cone against.
/// </param>
/// <param name="axisV">
/// Represents the "Y" axis to draw the base of the cone against.
/// </param>
/// <param name="radius">
/// Specifies the radius for the base of the cone.
/// </param>
/// <param name="length">
/// Specifies how tall the cone is.
/// </param>
/// <param name="color">
/// The color to use when drawing the cone.
/// </param>
private void DrawCone(Vector3 axis, Vector3 axisU, Vector3 axisV, float radius, float length, Color color)
{
GL.Begin(GL.TRIANGLES);
GL.Color(color);
// pre-calculate the angle step for each cone segment
var angle = (2 * Mathf.PI) / this.coneSegments;
for (var i = 0; i <= this.coneSegments; i++)
{
var pt = axisU * Mathf.Cos(angle * i) * radius;
pt += axisV * Mathf.Sin(angle * i) * radius;
pt += axis * length;
GL.Vertex(pt);
pt = axisU * Mathf.Cos(angle * (i + 1)) * radius;
pt += axisV * Mathf.Sin(angle * (i + 1)) * radius;
pt += axis * length;
GL.Vertex(pt);
GL.Vertex(axis);
}
GL.End();
}
/// <summary>
/// Uses OpenGL to draw a square plane for the axis.
/// </summary>
/// <param name="size">
/// Defines size of the plane that will be drawn for the axis.
/// </param>
/// <param name="axisU">
/// Represents the "X" axis to draw the plane against.
/// </param>
/// <param name="axisV">
/// Represents the "Y" axis to draw the plane against.
/// </param>
/// <param name="color">
/// The color to use when drawing the plane.
/// </param>
private void DrawQuad(float size, Vector3 axisU, Vector3 axisV, Color color)
{
color.a = this.planeTransparency;
var pts = new Vector3[4];
pts[0] = Vector3.zero;
pts[1] = axisU * size;
pts[2] = (axisU + axisV) * size;
pts[3] = axisV * size;
GL.Begin(GL.QUADS);
GL.Color(color);
GL.Vertex(pts[0]);
GL.Vertex(pts[1]);
GL.Vertex(pts[2]);
GL.Vertex(pts[3]);
GL.End();
color.a = 1f;
GL.Begin(GL.LINES);
GL.Color(color);
GL.Vertex(pts[0]);
GL.Vertex(pts[1]);
GL.Vertex(pts[1]);
GL.Vertex(pts[2]);
GL.Vertex(pts[2]);
GL.Vertex(pts[3]);
GL.Vertex(pts[3]);
GL.Vertex(pts[0]);
GL.End();
}
/// <summary>
/// Start is called just before any of the Update methods is called the first time.
/// </summary>
public void Start()
{
if (this.cameraReference == null)
{
this.cameraReference = UnityEngine.Camera.main;
}
}
/// <summary>
/// Handles dragging along the XU plane.
/// </summary>
/// <param name="ray">
/// The reay used to determine if the XY plane was being interacted with.
/// </param>
private void HandleXY(Ray ray)
{
float enter;
this.planeXY.Raycast(ray, out enter);
var hit = ray.GetPoint(enter);
hit = this.rotationMatrix.inverse.MultiplyPoint(hit);
if (this.typeMovementBeingMade == TypeOfMove.None && hit.x > 0f && hit.x <= this.panScale * this.cameraDistance && hit.y > 0
&& hit.y <= this.panScale * this.cameraDistance)
{
this.typeMovementBeingMade = TypeOfMove.XYAxis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.XYAxis)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.z = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
if (this.typeMovementBeingMade == TypeOfMove.None && hit.x > 0f && hit.x <= this.cameraDistance
&& Mathf.Abs(hit.y) < this.axisEnvelope * this.cameraDistance)
{
// case x
this.typeMovementBeingMade = TypeOfMove.X2Axis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.X2Axis && this.inputAxisChanged)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.y = 0;
this.hitMouseMove.z = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
if (this.typeMovementBeingMade == TypeOfMove.None && hit.y > 0f && hit.y <= this.cameraDistance
&& Mathf.Abs(hit.x) < this.axisEnvelope * this.cameraDistance)
{
// case y
this.typeMovementBeingMade = TypeOfMove.YAxis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.YAxis && this.inputAxisChanged)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.z = 0;
this.hitMouseMove.x = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
}
/// <summary>
/// Handles dragging along the XZ plane.
/// </summary>
/// <param name="ray">
/// The reay used to determine if the XZ plane was being interacted with.
/// </param>
private void HandleXZ(Ray ray)
{
float enter;
this.planeXZ.Raycast(ray, out enter);
var hit = ray.GetPoint(enter);
hit = this.rotationMatrix.inverse.MultiplyPoint(hit);
if (this.typeMovementBeingMade == TypeOfMove.None && hit.x > 0f && hit.x <= this.panScale * this.cameraDistance && hit.z > 0
&& hit.z <= this.panScale * this.cameraDistance)
{
this.typeMovementBeingMade = TypeOfMove.XZAxis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.XZAxis && this.inputAxisChanged)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.y = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
if (this.typeMovementBeingMade == TypeOfMove.None && hit.x > 0f && hit.x <= this.cameraDistance
&& Mathf.Abs(hit.z) < this.axisEnvelope * this.cameraDistance)
{
// case x
this.typeMovementBeingMade = TypeOfMove.XAxis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.XAxis && this.inputAxisChanged)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.y = 0;
this.hitMouseMove.z = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
if (this.typeMovementBeingMade == TypeOfMove.None && hit.z > 0f && hit.z <= this.cameraDistance
&& Mathf.Abs(hit.x) < this.axisEnvelope * this.cameraDistance)
{
// case z
this.typeMovementBeingMade = TypeOfMove.ZAxis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.ZAxis && this.inputAxisChanged)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.y = 0;
this.hitMouseMove.x = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
}
/// <summary>
/// Handles dragging along the YZ plane.
/// </summary>
/// <param name="ray">
/// The reay used to determine if the YZ plane was being interacted with.
/// </param>
private void HandleYZ(Ray ray)
{
float enter;
this.planeYZ.Raycast(ray, out enter);
var hit = ray.GetPoint(enter);
hit = this.rotationMatrix.inverse.MultiplyPoint(hit);
if (this.typeMovementBeingMade == TypeOfMove.None && hit.z > 0 && hit.z <= this.panScale * this.cameraDistance && hit.y > 0
&& hit.y <= this.panScale * this.cameraDistance)
{
this.typeMovementBeingMade = TypeOfMove.YZAxis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.YZAxis)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.x = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
if (this.typeMovementBeingMade == TypeOfMove.None && hit.z > 0f && hit.z <= this.cameraDistance
&& Mathf.Abs(hit.y) < this.axisEnvelope * this.cameraDistance)
{
// case z
this.typeMovementBeingMade = TypeOfMove.Z2Axis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.Z2Axis && this.inputAxisChanged)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.y = 0;
this.hitMouseMove.x = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
if (this.typeMovementBeingMade == TypeOfMove.None && hit.y > 0f && hit.y <= this.cameraDistance
&& Mathf.Abs(hit.z) < this.axisEnvelope * this.cameraDistance)
{
// case y
this.typeMovementBeingMade = TypeOfMove.Y2Axis;
this.hitMouseDown = hit;
this.isDragging = true;
}
if (this.typeMovementBeingMade == TypeOfMove.Y2Axis && this.inputAxisChanged)
{
this.hitMouseMove = hit - this.hitMouseDown;
this.hitMouseMove.z = 0;
this.hitMouseMove.x = 0;
this.UpdatePosition(this.worldTranslation ? this.hitMouseMove : this.transform.localRotation * this.hitMouseMove);
}
}
/// <summary>
/// Called by unity when after camera has rendered the scene.
/// </summary>
private void OnRenderObject()
{
// set the material pass is a material has been specified
var material = this.lineMaterial;
if (material != null)
{
material.SetPass(0);
}
// push a matrix on to the stack
GL.PushMatrix();
this.rotationMatrix = this.transform.localToWorldMatrix;
// setup rotation matrix
if (this.worldTranslation)
{
this.rotationMatrix = Matrix4x4.TRS(this.transform.position, Quaternion.identity, Vector3.one);
}
else
{
this.rotationMatrix = Matrix4x4.TRS(this.transform.position, this.transform.localRotation, Vector3.one);
}
GL.MultMatrix(this.rotationMatrix);
// draw each axis
this.DrawAxis(this.xAxisColor, Vector3.right, Vector3.up, Vector3.forward);
this.DrawAxis(this.yAxisColor, Vector3.up, Vector3.right, Vector3.forward);
this.DrawAxis(this.zAxisColor, Vector3.forward, Vector3.right, Vector3.up);
// draw each plane quad
this.DrawQuad(this.panScale * this.cameraDistance, Vector3.forward, Vector3.up, this.xAxisColor);
this.DrawQuad(this.panScale * this.cameraDistance, Vector3.right, Vector3.forward, this.yAxisColor);
this.DrawQuad(this.panScale * this.cameraDistance, Vector3.right, Vector3.up, this.zAxisColor);
GL.PopMatrix();
}
/// <summary>
/// Updates the transform position and raises the <see cref="PositionChanged"/> event.
/// </summary>
/// <param name="value">
/// The value to add on to the existing transform position.
/// </param>
private void UpdatePosition(Vector3 value)
{
var position = this.transform.position;
var previousPosition = position;
position += value;
this.transform.position = position;
this.OnPositionChanged(previousPosition, position);
}
#endregion
}
///
/// Provides event arguments for the type.
///
public class Vector3EventArgs : EventArgs
{
#region Constructors and Destructors
///
/// Initializes a new instance of the class.
///
///
/// The previous value.
///
///
/// The current value.
///
public Vector3EventArgs(Vector3 previousValue, Vector3 value)
{
this.Value = value;
this.PreviousValue = previousValue;
}
///
/// Initializes a new instance of the class.
///
public Vector3EventArgs()
{
}
#endregion
#region Public Properties
///
/// Gets or sets a previous value.
///
public Vector3 PreviousValue { get; set; }
///
/// Gets or sets a value.
///
public Vector3 Value { get; set; }
#endregion
}
bbdd826a-6708-4518-b651-d5214e52071e|3|4.7
I needed to add tab key support to a GUI.TextArea and soon discovered it was not quite as easy as I had originally thought. I have provided two code examples below. The first example is simplified and the second example is more complex that wraps TextArea controls inside of parent control.
With these examples you can type text in a TextArea/TextField and press the tab key to insert 4 spaces, or press Shift+Tab to move the line 4 spaces to the left if the area is clear.
I have also provided a third advanced example from my UIControls library to give an example of a real word usage scenario. This third example synchronizes my TextBox control with the unity’s TextEditor. My TextBox control has similar properties as Winforms TextBox.
Simplified example
using System;
using UnityEditor;
using UnityEngine;
public class TextAreaTabSupport : EditorWindow
{
private int lastKBFocus = -1;
private string textA = string.Empty;
private string textB = string.Empty;
private string textC = string.Empty;
[MenuItem("Test/Text Area Tab Support")]
public static void ShowWindow()
{
GetWindow<TextAreaTabSupport>().Show();
}
public void OnGUI()
{
var current = Event.current;
GUI.SetNextControlName("testa");
if (GUI.GetNameOfFocusedControl() == "testa" && this.lastKBFocus == GUIUtility.keyboardControl)
{
if (current.type == EventType.KeyDown || current.type == EventType.KeyUp)
{
if (current.isKey && (current.keyCode == KeyCode.Tab || current.character == '\t'))
{
if (current.type == EventType.KeyUp)
{
var te = (TextEditor)GUIUtility.GetStateObject(typeof(TextEditor), GUIUtility.keyboardControl);
if (!current.shift)
{
for (var i = 0; i < 4; i++)
{
te.Insert(' ');
}
}
else
{
var min = Math.Min(te.cursorIndex, te.selectIndex);
var index = min;
var temp = te.text;
for (var i = 1; i < 5; i++)
{
if ((min - i) < 0 || temp[min - i] != ' ')
{
break;
}
index = min - i;
}
if (index < min)
{
te.selectIndex = index;
te.cursorIndex = min;
te.ReplaceSelection(string.Empty);
}
}
this.textA = te.text;
}
current.Use();
}
}
}
this.textA = GUI.TextArea(new Rect(0, 40, 100, 100), this.textA);
if (GUI.GetNameOfFocusedControl() == "testa" && current.type == EventType.KeyDown || current.type == EventType.KeyUp)
{
this.lastKBFocus = GUIUtility.keyboardControl;
}
GUI.SetNextControlName("testb");
this.textB = GUI.TextArea(new Rect(110, 40, 100, 100), this.textB);
GUI.SetNextControlName("testc");
this.textC = GUI.TextField(new Rect(220, 40, 100, 30), this.textC);
if (GUI.Button(new Rect(10, 110, 50, 25), "Click"))
{
}
}
}
And a more complex example
using System;
using UnityEditor;
using UnityEngine;
public class TextAreaTabSupport : EditorWindow
{
private Vector2 scroll;
private int lastKBFocus = -1;
private string textA = string.Empty;
private string textB = string.Empty;
private string textC = string.Empty;
[MenuItem("Test/Text Area Tab Support")]
public static void ShowWindow()
{
GetWindow<TextAreaTabSupport>().Show();
}
public void OnGUI()
{
var current = Event.current;
GUI.SetNextControlName("scroller");
using (var scroll = new GUI.ScrollViewScope(new Rect(Vector2.zero, new Vector2(330, 150)), this.scroll, new Rect(Vector2.zero, new Vector2(330, 150))))
{
this.scroll = scroll.scrollPosition;
if (GUI.GetNameOfFocusedControl() == "testa" && this.lastKBFocus == GUIUtility.keyboardControl)
{
if (current.type == EventType.KeyDown || current.type == EventType.KeyUp)
{
if (current.isKey && (current.keyCode == KeyCode.Tab || current.character == '\t'))
{
if (current.type == EventType.KeyUp)
{
var te = (TextEditor)GUIUtility.GetStateObject(typeof(TextEditor), GUIUtility.keyboardControl);
if (!current.shift)
{
for (var i = 0; i < 4; i++)
{
te.Insert(' ');
}
}
else
{
var min = Math.Min(te.cursorIndex, te.selectIndex);
var index = min;
var temp = te.text;
for (var i = 1; i < 5; i++)
{
if ((min - i) < 0 || temp[min - i] != ' ')
{
break;
}
index = min - i;
}
if (index < min)
{
te.selectIndex = index;
te.cursorIndex = min;
te.ReplaceSelection(string.Empty);
}
}
this.textA = te.text;
}
current.Use();
}
}
}
using (new GUI.GroupScope(new Rect(0, 0, 110, 110)))
{
GUI.SetNextControlName("testa");
this.textA = GUI.TextArea(new Rect(0, 4, 100, 100), this.textA);
}
if (this.lastKBFocus != GUIUtility.keyboardControl && (current.type == EventType.KeyDown || current.type == EventType.KeyUp))
{
this.lastKBFocus = GUIUtility.keyboardControl;
}
GUI.SetNextControlName("testb");
this.textB = GUI.TextArea(new Rect(110, 40, 100, 100), this.textB);
GUI.SetNextControlName("testc");
this.textC = GUI.TextField(new Rect(220, 40, 100, 30), this.textC);
if (GUI.Button(new Rect(10, 110, 50, 25), "Click"))
{
}
}
}
}
Advanced example
namespace Codefarts.UIControls.Renderers
{
#if UNITY_5
using System;
using UnityEngine;
/// <summary>
/// Provides a renderer implementation for the <see cref="TextBox"/> control.
/// </summary>
[ControlRenderer(typeof(TextBox))]
public class TextBoxRenderer : BaseRenderer
{
/// <summary>
/// Implemented by inheritors to draw the actual control.
/// </summary>
/// <param name="args">The rendering argument information.</param>
/// <exception cref="System.ArgumentNullException">control</exception>
public override void DrawControl(ControlRenderingArgs args)
{
var textBox = (TextBox)args.Control;
// unity gui does not like null strings
var text = textBox.Text == null ? string.Empty : textBox.Text;
var maxLength = textBox.MaxLength == 0 ? int.MaxValue : textBox.MaxLength;
var rect = new Rect(textBox.Location + args.Offset, textBox.Size);
var hsbVisibility = textBox.HorizontalScrollBarVisibility;
var vsbVisibility = textBox.VerticalScrollBarVisibility;
var alwaysShowHorizontal = hsbVisibility == ScrollBarVisibility.Visible;
var alwaysShowVertical = vsbVisibility == ScrollBarVisibility.Visible;
KeyCode keyCode;
bool isDown;
bool isUp;
string controlName;
this.GetKeyInfoAndSetControlName(textBox, out keyCode, out isDown, out isUp, out controlName, false);
// get style and sync it up
var style = textBox.GetStyle(Control.ControlStyle, true, GUI.skin.textArea);
if (textBox.Font != null)
{
style.SetFontStyle(textBox.Font);
}
var current = Event.current;
int lastKBFocus;
textBox.Properties.TryGetValueCast(ControlDrawingHelpers.LastKeyboardControlID, out lastKBFocus, -1);
var selectionStart = textBox.SelectionStart;
var selectionLength = textBox.SelectionLength;
if (GUI.GetNameOfFocusedControl() == controlName && lastKBFocus == GUIUtility.keyboardControl)
{
var te = (TextEditor)GUIUtility.GetStateObject(typeof(TextEditor), GUIUtility.keyboardControl);
// sync textbox & texteditor selections
this.SyncTextBoxSelection(TextBox.TextBoxSelectionStartChanged, te, textBox);
this.SyncTextBoxSelection(TextBox.TextBoxSelectionLengthChanged, te, textBox);
// process tab key if nessary
text = this.HandleTabKeyPress(ref selectionStart, te, current, textBox, text);
selectionLength = Math.Abs(te.cursorIndex - te.selectIndex);
}
// draw the text area/field control
textBox.Text = this.DrawActualTextControl(textBox, text, rect, alwaysShowHorizontal, alwaysShowVertical, style, maxLength, controlName);
// check if we need to record last keyboard control id
if (GUI.GetNameOfFocusedControl() == controlName && lastKBFocus != GUIUtility.keyboardControl)
{
textBox.Properties[ControlDrawingHelpers.LastKeyboardControlID] = GUIUtility.keyboardControl;
}
// check is text selection changed and sync if nessary
if (textBox.SelectionStart != selectionStart)
{
textBox.SelectionStart = selectionStart;
}
if (textBox.SelectionLength != selectionLength)
{
textBox.SelectionLength = selectionLength;
}
// Handle key events
this.HandleKeyEventsAfterControlDrawn(controlName, keyCode, isDown, textBox, isUp);
// handle mouse enter & leave events
this.HandleMouseEvents(textBox);
}
private string HandleTabKeyPress(ref int selectionStart, TextEditor te, Event current, TextBox textBox, string text)
{
selectionStart = Math.Min(te.cursorIndex, te.selectIndex);
if (current.type == EventType.KeyDown || current.type == EventType.KeyUp)
{
if (current.isKey && (current.keyCode == KeyCode.Tab || current.character == '\t'))
{
// consume the tab key event before drawing the control
if (current.type == EventType.KeyUp && textBox.AcceptsTab)
{
if (!current.shift)
{
for (var i = 0; i < 4; i++)
{
te.Insert(' ');
}
}
else
{
var min = selectionStart;
var index = min;
var temp = te.text;
for (var i = 1; i < 5; i++)
{
if ((min - i) < 0 || temp[min - i] != ' ')
{
break;
}
index = min - i;
}
if (index < min)
{
te.selectIndex = index;
te.cursorIndex = min;
te.ReplaceSelection(string.Empty);
}
}
selectionStart = Math.Min(te.cursorIndex, te.selectIndex);
text = te.text;
}
current.Use();
}
}
return text;
}
private void SyncTextBoxSelection(string name, TextEditor editor, TextBox tb)
{
var props = tb.Properties;
if (props != null)
{
bool changed;
if (props.TryGetValueCast(name, out changed, false) && changed)
{
props[name] = false;
this.SetTextEditorSelection(editor, tb.SelectionStart, tb.SelectionLength);
}
}
}
private void SetTextEditorSelection(TextEditor editor, int start, int length)
{
if (editor.cursorIndex < editor.selectIndex)
{
editor.cursorIndex = start;
editor.selectIndex = start + length;
}
else
{
editor.selectIndex = start;
editor.cursorIndex = start + length;
}
}
protected virtual string DrawActualTextControl(TextBox textBox, string text, Rect rect, bool alwaysShowHorizontal, bool alwaysShowVertical,
GUIStyle style, int maxLength, string controlName)
{
var scrollPosition = new Vector2(-textBox.HorizontalOffset, -textBox.VerticalOffset);
if (textBox.AcceptsReturn)
{
var textSize = GUI.skin.textArea.CalcSize(new GUIContent(text));
var viewRect = new Rect(Vector2.zero, textSize);
viewRect.width = Math.Max(textSize.x, rect.width);
viewRect.height = Math.Max(textSize.y, rect.height);
var drawHorizScroll = viewRect.width > rect.width || alwaysShowHorizontal;
var drawVertScroll = viewRect.height > rect.height || alwaysShowVertical;
var horizRect = new Rect(
0,
rect.height - GUI.skin.horizontalScrollbar.fixedHeight,
rect.width - (drawVertScroll ? GUI.skin.verticalScrollbar.fixedWidth : 0),
GUI.skin.horizontalScrollbar.fixedHeight);
var vertRect = new Rect(
rect.width - GUI.skin.verticalScrollbar.fixedWidth,
0,
GUI.skin.verticalScrollbar.fixedWidth,
rect.height - (drawHorizScroll ? GUI.skin.horizontalScrollbar.fixedHeight : 0));
horizRect.position += rect.position;
vertRect.position += rect.position;
scrollPosition.x = drawHorizScroll ? scrollPosition.x : 0;
scrollPosition.y = drawVertScroll ? scrollPosition.y : 0;
viewRect.position = scrollPosition;
var grpRect = new Rect(
rect.x,
rect.y,
rect.width - (drawVertScroll ? GUI.skin.verticalScrollbar.fixedWidth : 0),
rect.height - (drawHorizScroll ? GUI.skin.horizontalScrollbar.fixedHeight : 0));
using (new GUI.GroupScope(grpRect))
{
// draw background
var brush = textBox.Background;
if (brush != null)
{
BrushExtensions.Draw(brush, new Rect(Vector2.zero, grpRect.size));
}
GUI.SetNextControlName(controlName);
// as of unity v5.3 there is a bug that prevent me from specifying a maxlength
//BUG: see details here -> https://fogbugz.unity3d.com/default.asp?768436_vikdrmh7ernh03ls
text = GUI.TextArea(viewRect, text, style);
}
if (drawHorizScroll)
{
textBox.HorizontalOffset = GUI.HorizontalScrollbar(
horizRect,
textBox.HorizontalOffset,
Math.Min(viewRect.width, rect.width),
0,
viewRect.width + (drawVertScroll ? GUI.skin.verticalScrollbar.fixedWidth : 0));
}
if (drawVertScroll)
{
textBox.VerticalOffset = GUI.VerticalScrollbar(
vertRect,
textBox.VerticalOffset,
Math.Min(viewRect.height, rect.height),
0,
viewRect.height + (drawHorizScroll ? GUI.skin.horizontalScrollbar.fixedHeight : 0));
}
}
else
{
// draw background
var brush = textBox.Background;
if (brush != null)
{
BrushExtensions.Draw(brush, rect);
}
GUI.SetNextControlName(controlName);
text = GUI.TextField(rect, text, maxLength, style);
}
return text;
}
}
#endif
}
7e8e00a5-cad7-4eca-8192-9fb6363764d9|1|1.0
The class below provides a progress model for reporting the progress of an action. /// <summary>
/// Provides a progress class for reporting progress data.
/// </summary>
/// <typeparam name="T">The type used for the result data.</typeparam>
public class ProgressModel<T>
{
/// <summary>
/// Gets the results queue.
/// </summary>
public Queue<T> Results { get; private set; }
/// <summary>
/// Gets the message queue.
/// </summary>
public Queue<string> Messages { get; private set; }
/// <summary>
/// Gets or sets a value indicating whether this instance is completed.
/// </summary>
public bool IsComplete { get; set; }
/// <summary>
/// Flags the response model as canceled.
/// </summary>
public void Cancel()
{
this.IsCanceled = true;
}
/// <summary>
/// Initializes a new instance of the <see cref="ProgressModel{T}"/> class.
/// </summary>
public ProgressModel()
{
this.Results = new Queue<T>();
this.Messages = new Queue<string>();
}
/// <summary>
/// Gets a value indicating whether this instance is canceled.
/// </summary>
public bool IsCanceled { get; private set; }
/// <summary>
/// Gets or sets the progress.
/// </summary>
public float Progress { get; set; }
/// <summary>
/// Gets or sets the information that may have been thrown.
/// </summary>
public Exception Exception { get; set; }
/// <summary>
/// Gets a value indicating whether this instance is faulted.
/// </summary>
public bool IsFaulted
{
get
{
return this.Exception != null;
}
}
/// <summary>
/// Gets or sets the title.
/// </summary>
public string Title { get; set; }
}
8049ff95-cebf-4d09-af67-ded756539755|0|.0
The code below provides a handy helper method for drawing GUI textures via GUI.DrawTextureWithTexCoords.
///
/// Draws a image image.
///
/// The destination image.
/// The x position in the destination image.
/// The y position in the destination image.
/// The destination width of the drawn image.
/// The destination height of the drawn image.
/// If set to true the image will be drawn fliped horizontally.
/// If set to true the image will be drawen flipped vertically.
/// If set to true the image will be tiled across the destination area.
/// image
/// If width, height, sourceWidth or sourceHeight are less then 1.
public static void Draw(Texture2D image, float x, float y, float width, float height, bool flipHorizontally, bool flipVertically, bool tile)
{
Draw(image, x, y, width, height, 0, 0, image.width, image.height, false, false, false);
}
///
/// Draws a image image.
///
/// The destination image.
/// The x position in the destination image.
/// The y position in the destination image.
/// The destination width of the drawn image.
/// The destination height of the drawn image.
/// The x position in the source image.
/// The y position in the source image.
/// The source width.
/// The source height.
/// image
/// If width, height, sourceWidth or sourceHeight are less then 1.
public static void Draw(Texture2D image, float x, float y, float width, float height, float sourceX, float sourceY, float sourceWidth, float sourceHeight)
{
Draw(image, x, y, width, height, sourceX, sourceY, sourceWidth, sourceHeight, false, false, false);
}
///
/// Draws a image image.
///
/// The destination image.
/// The x position in the destination image.
/// The y position in the destination image.
/// The destination width of the drawn image.
/// The destination height of the drawn image.
/// The x position in the source image.
/// The y position in the source image.
/// The source width.
/// The source height.
/// If set to true the image will be drawn fliped horizontally.
/// If set to true the image will be drawen flipped vertically.
/// If set to true the image will be tiled across the destination area.
/// image
/// If width, height, sourceWidth or sourceHeight are less then 1.
/// This method has issues with Clamped textures.
public static void Draw(Texture2D image, float x, float y, float width, float height, float sourceX, float sourceY, float sourceWidth, float sourceHeight,
bool flipHorizontally, bool flipVertically, bool tile)
{
// perform input validation
if (image == null)
{
throw new ArgumentNullException("image");
}
if (sourceWidth < float.Epsilon)
{
throw new ArgumentOutOfRangeException("sourceWidth");
}
if (sourceHeight < float.Epsilon)
{
throw new ArgumentOutOfRangeException("sourceHeight");
}
if (width < float.Epsilon)
{
return;
}
if (height < float.Epsilon)
{
return;
}
var imgWidth = (float)image.width;
var imgHeight = (float)image.height;
var srcWidth = sourceWidth / imgWidth;
var srcHeight = sourceHeight / imgHeight;
var position = new Rect(x, y + height, width, -height);
if (tile)
{
srcWidth = width / imgWidth;
srcHeight = height / imgHeight;
}
srcWidth = flipHorizontally ? -srcWidth : srcWidth;
srcHeight = !flipVertically ? -srcHeight : srcHeight;
var texCoords = new Rect(sourceX / imgWidth, imgHeight - (sourceY / imgHeight), srcWidth, srcHeight);
GUI.DrawTextureWithTexCoords(position, image, texCoords, true);
}
92650749-1936-4014-b502-fc2e53082029|0|.0
|
|