I have been wondering how my Compaq nx9420 holds up to the Microsoft Surface Pro as I have been contemplating purchasing one. Even though it’s pushing 6 or 7 years old now my nx9420 is still a decent machine to work with Unity3D, Visual Studio, and to play older games on but the battery no longer holds a charge, the 5200 rpm 100Gb hard drive slows it down a lot compared to the 256Gb Samsung 830 SSD (replaced by the 840 series) I recently purchased for my desktop.
It’s at a point where given it’s age I feel it’s too old to sell, especially when you can get a decent machine for like $600 now a days. And I am hesitant to put any more money into it like a new battery and a ssd to try and extend it’s life a little more.
So I started to crunch some numbers and look around for performance comparisons I could do and I have documented the few comparisons I have done below.
Based off the Window Experience Index numbers alone it seems comparable to a Surface Pro, but after running the peacemaker browser benchmark there started to be a noticeable performance gap. Running PCMark7 there was a huge performance gap. I know the surface pro is more powerful but I am still trying to get a sense of just how much more powerful it actually is compared to my nx9420.
My laptop used to be my main machine for a number of years until I bought my desktop, And even though I hardly ever use my laptop anymore it sure is nice to have a mobile computer to pack around on long trips and when I am away from home.
The single biggest reason I am holding off getting a Surface Pro is the fact I cannot replace the battery. Even after 6+ years I can still buy a new $40 battery for my nx9420 off eBay. Given that I rarely have a use for a portable machine I will undoubtedly have the Surface Pro for a good 6+ years and if I can’t replace the battery, it no longer becomes a portable device seeing as how it will need to be plugged in all the time. Thus defeating the purpose of a mobile computer.
So without further adieu lets compare numbers shall we !
Windows Experience Index numbers are as follows. Keep in mind the math play below is simply a numerical comparison on the windows experience index numbers alone and does not reflect actual hardware features like the nx9420’s x1600 graphics with DX9 v2 vertex & pixel shader support compared to the HD4000’s (I’m guessing) DX11 v3 or v4 vertex & pixel shader support.
Win 7 experience index 1.0 to 7.9
Win 8 experience index 1.0 to 9.9
7.9 / 9.9 * 100 - 100 = 20.20% diff
Microsoft Surface Pro
Processor: 6.9 / 9.9 * 100 = 69.9% (core i5 third gen)
Memory: 5.9 / 9.9 * 100 = 59.59% (4gb)
Graphics: 5.6 / 9.9 * 100 = 56.56%
Gaming: 6.4 / 9.9 * 100 = 64.64% (HD4000 integrated)
Hard Disk: 8.1 / 9.9 * 100 = 81.81% (ssd)
Compaq nx9420
Processor: 3.7 / 7.9 * 100 = 46.83% (Core i2 dual core T7200 2.0Ghz)
Memory: 4.4 / 7.9 * 100 = 55.69% (4gb but intel chipset only recognizes 3gb)
Graphics: 4.2 / 7.9 * 100 = 51.16%
Gaming: 4.3 / 7.9 * 100 = 54.43% (ati x1600 discrete)
Hard Disk: 4.4 / 7.9 * 100 = 55.69% (hdd 5200rpm)
nx9420 Win7 Experience Index scaled values to Win8 Experience Index (0.202 = 20.20%)
Processor: 46.83% * 0.202 = 9.45% adjusted result is 56.28%
Memory: 55.69% * 0.202 = 11.24% adjusted result is 66.93%
Graphics: 51.16% * 0.202 = 10.33% adjusted result is 61.49%
Gaming: 54.43% * 0.202 = 10.99% adjusted result is 65.42%
Hard Disk: 55.69% * 0.202 = 11.24% adjusted result is 66.93%
nx9420 verses Surface Pro relative windows experience index
Processor: 56.28% -> 69.9% = -13.2% slower
Memory: 66.93% -> 59.59% = +7.34% faster
Graphics: 61.49% -> 56.56% = +4.93% faster
Gaming: 65.42% -> 64.64% = +0.78% faster
Hard Disk: 66.93% -> 81.81% = -14.88% slower
Compared the the performance numbers from the other tests listed below if I was to compare my nx9420 to the Surface Pro based on the windows experience index numbers alone one could get the false impression that the Surface Pro is not that much of a upgrade. Memory, Graphics and Gaming numbers are almost identical when comparing the Win 7 Experience index numbers relative to Win 8’s Experience index numbers.
Microsoft Surface Pro IE10
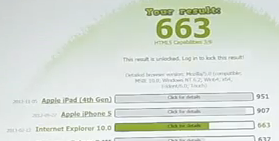
nx9420 Laptop Chrome v24.0
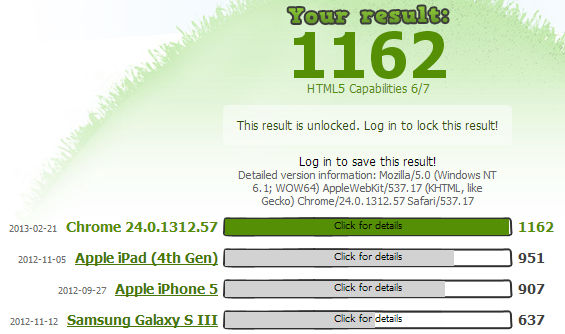
nx9420 Laptop IE9 64bit
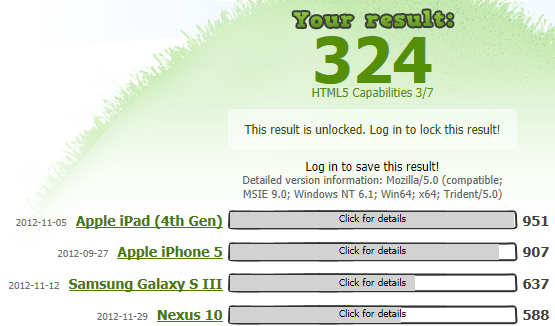
nx9420 Laptop IE9 32bit
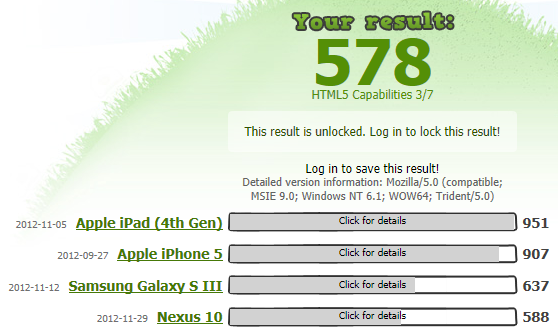
nx9420 Laptop Firefox 19
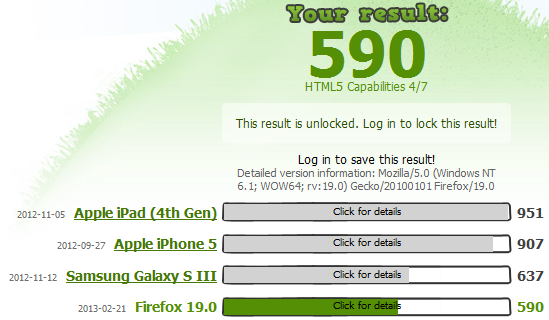
My desktop win 8 pro (IE10 desktop mode 32bit)
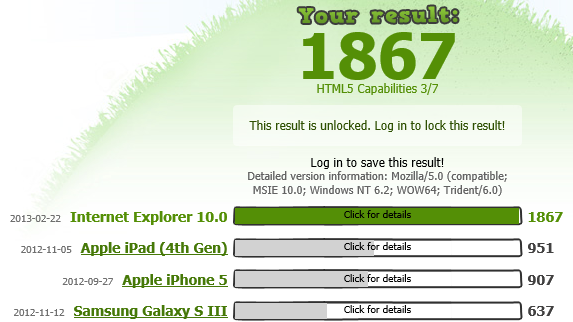
My desktop win 8 pro Firefox 19
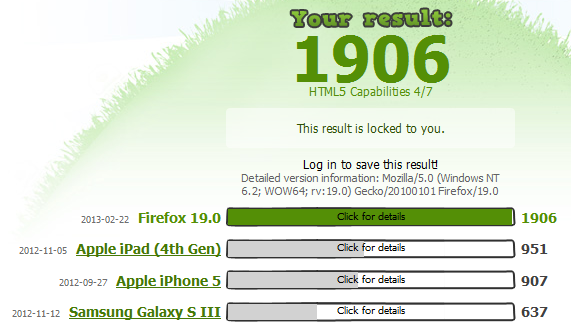
Microsoft Surface Pro Score: 4657 (paid version)
Source –> MobleTechReview website
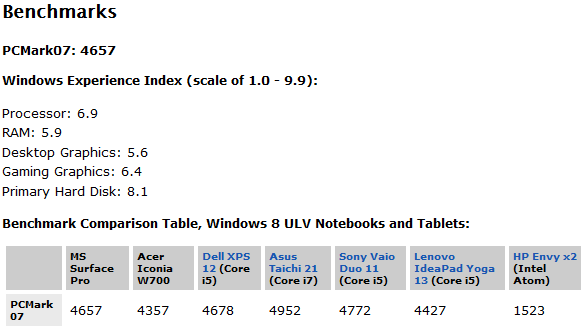
Compaq nx9420 Score 844 (free version)
Link to the results are here –> http://www.3dmark.com/pcm7/569200
DOTA 2 results
The Youtube channel SurfaceProGaming has a number of games that show the gaming performance of the Surface Pro.
Dota 2 on Surface Pro runs at about an average of say 30 fps at resolution of 1280x720 (921,600 pixels) while recording fraps.
Using the same graphics settings with the closest resolution my nx9420 lets me select 1024x768 (786,432 pixels) I can run DOTA 2 at an average of 15 to 25 fps without recording fraps. Because of the 5200 rpm HDD is 80% full I only get 5 to 8 fps when fraps is recording.
In Conclusion
When comparing the Surface Pro to my nx9420 running DOTA 2 the Surface Pro has a noticeable increase in capability but in my opinion not enough to justify getting one. The fact that my nx9420 is 6+ years old and still “somewhat” keeps up with the Surface Pro in DOTA 2 is a strong indicator that my nx9420 is still a fairly decent system for what I use it for.
I don’t play too many games on my laptop other then simple games like Angry Birds, The Binding of Issac and older games like the legacy of kain series of games that I recently played on my laptop. For larger games like Starcraft 2, World of Warcraft, Skyrim, Crysis etc I will always play on my desktop.
If the Surface Pro showed a more drastic performance in DOTA 2 AND had a removable battery I would have given some serious thought into getting a Surface Pro but that’s not goanna happen after running these comparisons. Investing in a 128Gb SSD and a new battery for my nx9420 is a far more viable solution economically and strategically because after I decide to sell or retire my laptop I can always add the SDD to my desktop and a new battery is cheap at around $40.
I had also looked into the Razer Edge Pro but although it’s more graphically capable then the Surface Pro again it too has no removable battery, and a lower resolution the then Surface Pro, and no stylus pen.
My next portable computer will be a tablet but only if it has a discrete graphics chip, pen input, and a removable battery. I think I may be waiting quite a few years for that to happen.
Hope this gives some people a sense of how much of an upgrade buying a Surface Pro would be compared to using a older laptop.