With the introduction to Unity 5 there comes some api changes. Specifically this foot note was interesting “[2] in Unity5 we also cache the transform component on the c# side, so there should no longer be a performance reason to cache the transform component yourself.”
I decided to test it out by writing a few performance test scripts and comparing performance numbers. Below is a screen shot of my results along with the scripts used.
As you can see caching a reference to the transform component in the Start method then using that reference is still faster then calling “this.transform” directly albeit only slightly by about 10-20 ticks. And calling “this.GetComponent<Transform>()” is almost twice as slow.
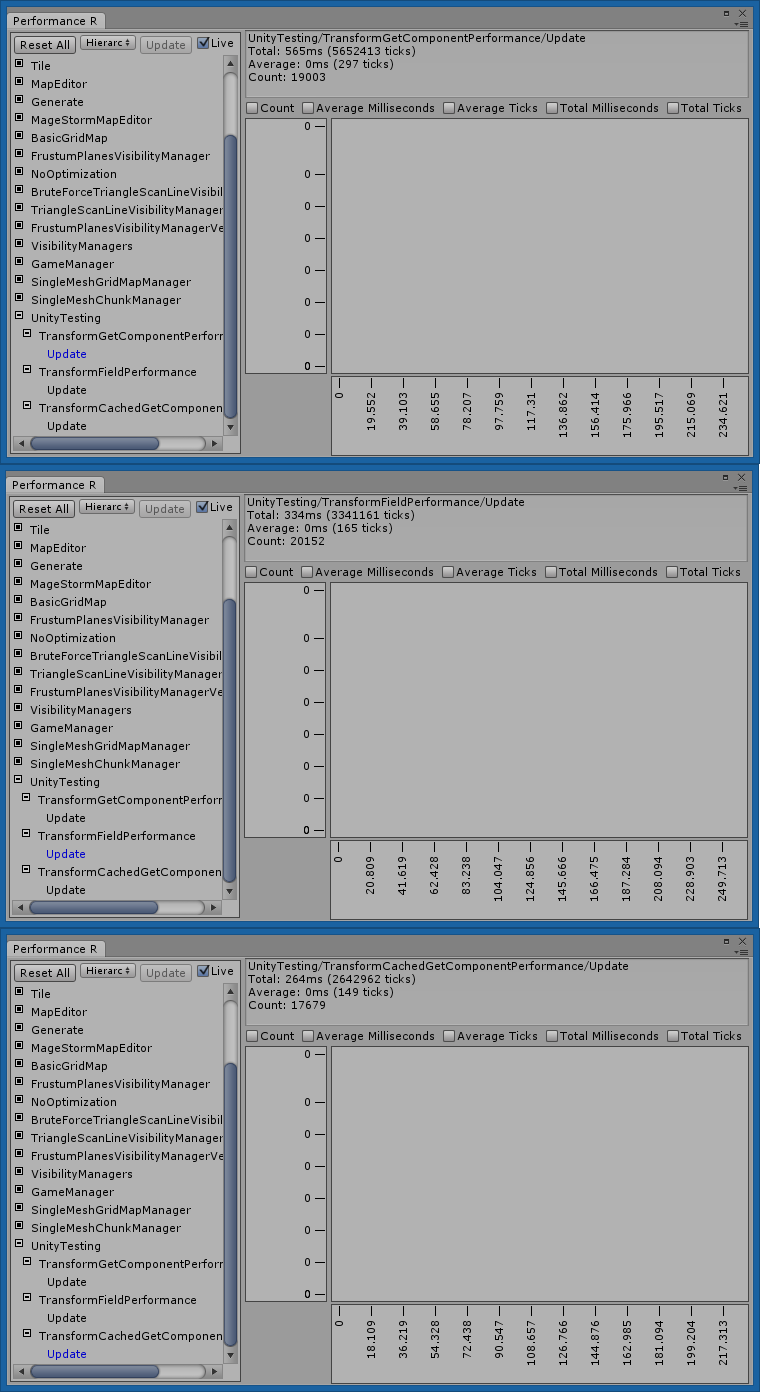
The code for the PerformanceTesting class is availible here.
TransformCachedGetComponentPerformance script
using UnityEngine;
public class TransformCachedGetComponentPerformance : MonoBehaviour
{
private Transform reference;
/// <summary>
/// Awake is called when the script instance is being loaded.
/// </summary>
public void Awake()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Create("UnityTesting/TransformCachedGetComponentPerformance/Update");
#endif
}
/// <summary>
/// Start is called just before any of the Update methods is called the first time.
/// </summary>
public void Start()
{
this.reference = this.GetComponent<Transform>();
}
/// <summary>
/// Update is called every frame, if the MonoBehaviour is enabled.
/// </summary>
public void Update()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Start("UnityTesting/TransformCachedGetComponentPerformance/Update");
#endif
var rnd = new System.Random();
this.reference.localPosition = new Vector3(rnd.Next(-3, 3), rnd.Next(-3, 3), rnd.Next(-3, 3));
#if PERFORMANCE
perf.Stop("UnityTesting/TransformCachedGetComponentPerformance/Update");
#endif
}
}
TransformGetComponentPerformance script
using UnityEngine;
public class TransformGetComponentPerformance : MonoBehaviour
{
/// <summary>
/// Awake is called when the script instance is being loaded.
/// </summary>
public void Awake()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Create("UnityTesting/TransformGetComponentPerformance/Update");
#endif
}
/// <summary>
/// Update is called every frame, if the MonoBehaviour is enabled.
/// </summary>
public void Update()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Start("UnityTesting/TransformGetComponentPerformance/Update");
#endif
var rnd = new System.Random();
this.GetComponent<Transform>().localPosition = new Vector3(rnd.Next(-3, 3), rnd.Next(-3, 3), rnd.Next(-3, 3));
#if PERFORMANCE
perf.Stop("UnityTesting/TransformGetComponentPerformance/Update");
#endif
}
}
TransformFieldPerformance script
using UnityEngine;
public class TransformFieldPerformance : MonoBehaviour
{
/// <summary>
/// Awake is called when the script instance is being loaded.
/// </summary>
public void Awake()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Create("UnityTesting/TransformFieldPerformance/Update");
#endif
}
/// <summary>
/// Update is called every frame, if the MonoBehaviour is enabled.
/// </summary>
public void Update()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Start("UnityTesting/TransformFieldPerformance/Update");
#endif
var rnd = new System.Random();
this.transform.localPosition = new Vector3(rnd.Next(-3, 3), rnd.Next(-3, 3), rnd.Next(-3, 3));
#if PERFORMANCE
perf.Stop("UnityTesting/TransformFieldPerformance/Update");
#endif
}
}