Calendar
Mo | Tu | We | Th | Fr | Sa | Su |
---|
30 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | 29 | 30 | 31 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
Archive
- 2002
- 2003
- 2004
- 2005
- 2006
- 2007
- 2008
- 2009
- 2010
- 2011
- 2012
- 2013
- 2014
- 2015
- 2016
- 2019
- 2020
|
Website may be up and down over next few months. I'm currently doing a complete overhaul of everything.
Going back to simple individual .htm pages, new overall site theme, sanitizing and cleaning up html of all
pages and blog posts, attempting to implement a new tooling and publishing system etc etc.
Takes in an array of floats representing pixels ordered left to right top to bottom. Width & height arguments specify the image dimensions. /// <summary>
/// Smoothens out the image.
/// </summary>
/// <param name="image">The source image.</param>
/// <exception cref="ArgumentNullException"><paramref name="getPixel"/> or <paramref name="setPixel"/> is <see langword="null" />.</exception>
public static void Smoothen(this float[] image, int width, int height, Func<int, int, float> getPixel, Action<int, int, float> setPixel, float smoothinValue = 9f)
{
if (getPixel == null)
{
throw new ArgumentNullException("getPixel");
}
if (setPixel == null)
{
throw new ArgumentNullException("setPixel");
}
for (var x = 1; x < width - 1; ++x)
{
for (var y = 1; y < height - 1; ++y)
{
var total = 0f;
for (var u = -1; u <= 1; u++)
{
for (var v = -1; v <= 1; v++)
{
total += getPixel(x + u, y + v);
}
}
setPixel(x, y, total / smoothinValue);
}
}
}
f23c8083-231a-402e-8106-dfb0d004f568|0|.0
The fallowing script allows you to control fog settings on a per camera basis, allowing you to use say green fog for one camera but red fog for another camera.
Unity 5 package demo is available here CameraFog.unitypackage (46.66 kb)
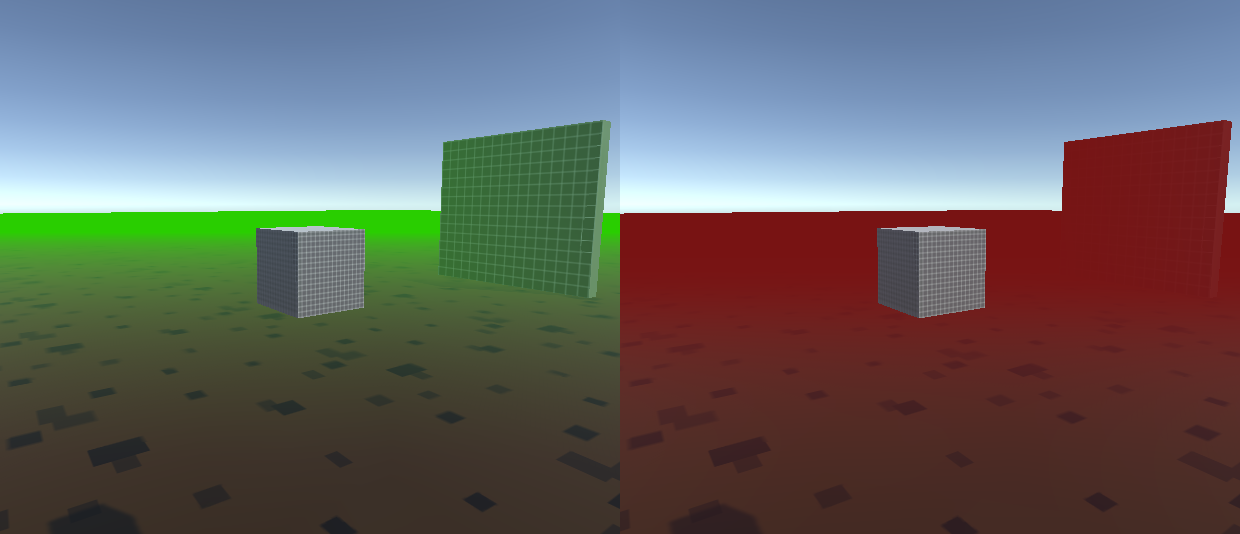
CameraFog.cs script
// --------------------------------------------------------------------------------------------------------------------
// <copyright file="CameraFog.cs" company="Codefarts">
// Copyright (c) 2012 Codefarts
// All rights reserved.
// contact@codefarts.com
// http://www.codefarts.com
// </copyright>
// --------------------------------------------------------------------------------------------------------------------
// Per-camera fog
// This is a simple class that, when added to a GameObject with a camera, allows you to control the fog settings for that camera separately from the global ones.
// I'd love to hear from you if you do anything cool with this or have any suggestions :)
// Original author: http://wiki.unity3d.com/index.php/User:Tenebrous
// Author website as of 2015: www.tenebrous.co.uk
// Source: http://wiki.unity3d.com/index.php/CameraFog
namespace Codefarts.GeneralTools.Scripts.Camera
{
using UnityEngine;
/// <summary>
/// Modifies a camera to allows you to control the fog settings for that camera separately from the global scene fog or other cameras.
/// </summary>
[RequireComponent(typeof(Camera))]
[ExecuteInEditMode]
public class CameraFog : MonoBehaviour
{
/// <summary>
/// The enabled state weather or not fog will be visible.
/// </summary>
public bool Enabled;
/// <summary>
/// The start distance from the camera where the fog will be drawn.
/// </summary>
public float StartDistance;
/// <summary>
/// The end distance from the camera where the fog will be drawn.
/// </summary>
public float EndDistance;
/// <summary>
/// The fog mode that controls how the fog is rendered.
/// </summary>
public FogMode Mode;
/// <summary>
/// The density of the fog that is rendered.
/// </summary>
public float Density;
/// <summary>
/// The fog color.
/// </summary>
public Color Color;
/// <summary>
/// Stores the pre-render state of the start distance.
/// </summary>
private float _startDistance;
/// <summary>
/// Stores the pre-render state of the end distance.
/// </summary>
private float _endDistance;
/// <summary>
/// Stores the pre-render state of the fog mode.
/// </summary>
private FogMode _mode;
/// <summary>
/// Stores the pre-render state of the density.
/// </summary>
private float _density;
/// <summary>
/// Stores the pre-render state of the fog color.
/// </summary>
private Color _color;
/// <summary>
/// Stores the pre-render state wheather or not the fog is enabled.
/// </summary>
private bool _enabled;
/// <summary>
/// Event that is fired before any camera starts rendering.
/// </summary>
private void OnPreRender()
{
this._startDistance = RenderSettings.fogStartDistance;
this._endDistance = RenderSettings.fogEndDistance;
this._mode = RenderSettings.fogMode;
this._density = RenderSettings.fogDensity;
this._color = RenderSettings.fogColor;
this._enabled = RenderSettings.fog;
RenderSettings.fog = this.Enabled;
RenderSettings.fogStartDistance = this.StartDistance;
RenderSettings.fogEndDistance = this.EndDistance;
RenderSettings.fogMode = this.Mode;
RenderSettings.fogDensity = this.Density;
RenderSettings.fogColor = this.Color;
}
/// <summary>
/// Event that is fired after any camera finishes rendering.
/// </summary>
private void OnPostRender()
{
RenderSettings.fog = this._enabled;
RenderSettings.fogStartDistance = this._startDistance;
RenderSettings.fogEndDistance = this._endDistance;
RenderSettings.fogMode = this._mode;
RenderSettings.fogDensity = this._density;
RenderSettings.fogColor = this._color;
}
}
}
3e68139e-e598-4f81-95a0-97e5ff0eeec9|0|.0
With the introduction to Unity 5 there comes some api changes. Specifically this foot note was interesting “[2] in Unity5 we also cache the transform component on the c# side, so there should no longer be a performance reason to cache the transform component yourself.”
I decided to test it out by writing a few performance test scripts and comparing performance numbers. Below is a screen shot of my results along with the scripts used.
As you can see caching a reference to the transform component in the Start method then using that reference is still faster then calling “this.transform” directly albeit only slightly by about 10-20 ticks. And calling “this.GetComponent<Transform>()” is almost twice as slow.
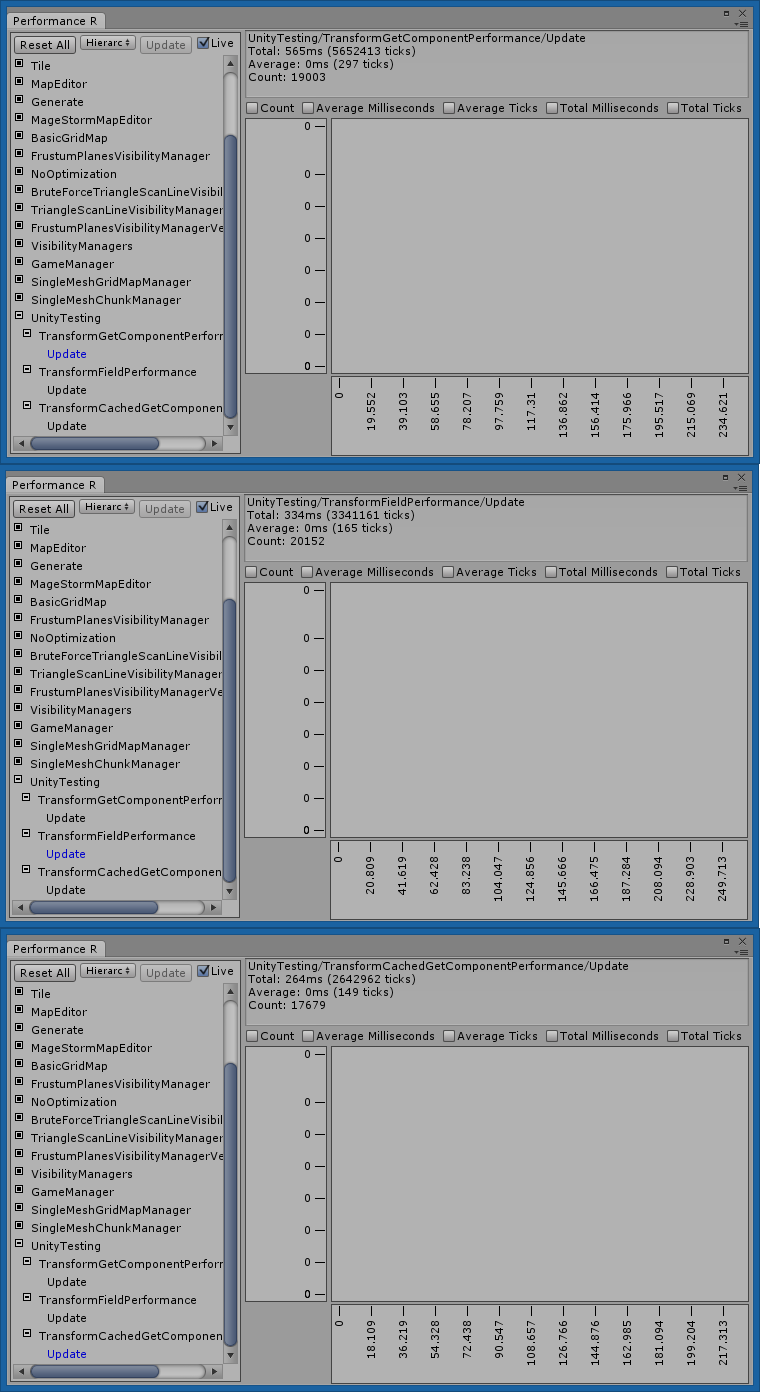
The code for the PerformanceTesting class is availible here.
TransformCachedGetComponentPerformance script
using UnityEngine;
public class TransformCachedGetComponentPerformance : MonoBehaviour
{
private Transform reference;
/// <summary>
/// Awake is called when the script instance is being loaded.
/// </summary>
public void Awake()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Create("UnityTesting/TransformCachedGetComponentPerformance/Update");
#endif
}
/// <summary>
/// Start is called just before any of the Update methods is called the first time.
/// </summary>
public void Start()
{
this.reference = this.GetComponent<Transform>();
}
/// <summary>
/// Update is called every frame, if the MonoBehaviour is enabled.
/// </summary>
public void Update()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Start("UnityTesting/TransformCachedGetComponentPerformance/Update");
#endif
var rnd = new System.Random();
this.reference.localPosition = new Vector3(rnd.Next(-3, 3), rnd.Next(-3, 3), rnd.Next(-3, 3));
#if PERFORMANCE
perf.Stop("UnityTesting/TransformCachedGetComponentPerformance/Update");
#endif
}
}
TransformGetComponentPerformance script
using UnityEngine;
public class TransformGetComponentPerformance : MonoBehaviour
{
/// <summary>
/// Awake is called when the script instance is being loaded.
/// </summary>
public void Awake()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Create("UnityTesting/TransformGetComponentPerformance/Update");
#endif
}
/// <summary>
/// Update is called every frame, if the MonoBehaviour is enabled.
/// </summary>
public void Update()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Start("UnityTesting/TransformGetComponentPerformance/Update");
#endif
var rnd = new System.Random();
this.GetComponent<Transform>().localPosition = new Vector3(rnd.Next(-3, 3), rnd.Next(-3, 3), rnd.Next(-3, 3));
#if PERFORMANCE
perf.Stop("UnityTesting/TransformGetComponentPerformance/Update");
#endif
}
}
TransformFieldPerformance script
using UnityEngine;
public class TransformFieldPerformance : MonoBehaviour
{
/// <summary>
/// Awake is called when the script instance is being loaded.
/// </summary>
public void Awake()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Create("UnityTesting/TransformFieldPerformance/Update");
#endif
}
/// <summary>
/// Update is called every frame, if the MonoBehaviour is enabled.
/// </summary>
public void Update()
{
#if PERFORMANCE
var perf = PerformanceTesting.PerformanceTesting<string>.Instance;
perf.Start("UnityTesting/TransformFieldPerformance/Update");
#endif
var rnd = new System.Random();
this.transform.localPosition = new Vector3(rnd.Next(-3, 3), rnd.Next(-3, 3), rnd.Next(-3, 3));
#if PERFORMANCE
perf.Stop("UnityTesting/TransformFieldPerformance/Update");
#endif
}
}
0631a2dd-08a4-4b60-b9fa-0f8221b4adb9|2|5.0
The fallowing code snip is designed to take in a flat list of file paths (or similar data) and produce a hierarchy of tree nodes representing those file paths.
/// <summary>
/// Constructs a nested hierarchy of types from a flat list of source types.
/// </summary>
/// <typeparam name="TSource">The source type of the flat list that is to be converted.</typeparam>
/// <typeparam name="TReturn">The type that will be returned.</typeparam>
/// <typeparam name="TPart">The type of the art type.</typeparam>
/// <param name="sourceItems">The source items to be converted.</param>
/// <param name="getParts">A callback function that returns a array of <see cref="TPart"/>.</param>
/// <param name="comparePart">The compare part callback.</param>
/// <param name="getChildren">The get children callback.</param>
/// <param name="addChild">The add child callback.</param>
/// <param name="createItem">The create item callback.</param>
/// <returns>Returns an collection of <see cref="TReturn"/> representing the hierarchy.</returns>
/// <exception cref="Exception">A delegate callback throws an exception. </exception>
private static IEnumerable<TReturn> ToHierarchy<TSource, TReturn, TPart>(
IEnumerable<TSource> sourceItems,
Func<TSource, TPart[]> getParts,
Func<TReturn, TPart, bool> comparePart,
Func<TReturn, IEnumerable<TReturn>> getChildren,
Action<IEnumerable<TReturn>, TReturn> addChild,
Func<TPart[], int, TSource, TReturn> createItem)
{
var treeModels = new List<TReturn>();
foreach (var keyName in sourceItems)
{
IEnumerable<TReturn> items = treeModels;
var parts = getParts(keyName);
for (var partIndex = 0; partIndex < parts.Length; partIndex++)
{
var node = items.FirstOrDefault(x => comparePart(x, parts[partIndex]));
if (node != null)
{
items = getChildren(node);
continue;
}
var model = createItem(parts, partIndex, keyName);
addChild(items, model);
items = getChildren(model);
}
}
return treeModels;
}
An example of how one could use the ToHierarchy method would be like this …
var separator = new[] { Path.AltDirectorySeparatorChar.ToString(CultureInfo.InvariantCulture) };
// paths varible could be something from Directory.GetDirectories method for example.
var nodes = ToHierarchy<string, TreeViewNode, string>(
paths.OrderBy(x => x),
x => x.Split(separator, StringSplitOptions.RemoveEmptyEntries),
(r, p) => string.CompareOrdinal(r.Name, p) == 0,
r => r.Nodes,
(r, c) => ((List<TreeViewNode>)r).Add(c),
this.CreateTreeNode);
private TreeViewNode CreateTreeNode(string[] parts, int index, string source)
{
var node = new TreeViewNode() { Name = parts[index] };
node.Value = string.Join(Path.DirectorySeparatorChar.ToString(CultureInfo.InvariantCulture), parts, 0, index + 1);
if (index == parts.Length - 1)
{
node.Name = Path.GetFileName(source);
}
node.IsFile = File.Exists(node.Value);
return node;
}
Where paths is a array of file paths from say Directory.GetFiles.
3bebbb17-378e-4ffb-b2dd-e42a8fb57745|0|.0
Source: http://stackoverflow.com/questions/616718/how-do-i-get-common-file-type-icons-in-c public static class FileIcon
{
[DllImport("shell32.dll")]
private static extern IntPtr SHGetFileInfo(string pszPath, uint dwFileAttributes, ref SHFILEINFO psfi, uint cbSizeFileInfo, uint uFlags);
[StructLayout(LayoutKind.Sequential)]
private struct SHFILEINFO
{
public IntPtr hIcon;
public IntPtr iIcon;
public uint dwAttributes;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 260)]
public string szDisplayName;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 80)]
public string szTypeName;
};
private const uint SHGFI_ICON = 0x100;
private const uint SHGFI_LARGEICON = 0x0; // 'Large icon
private const uint SHGFI_SMALLICON = 0x1; // 'Small icon
public static System.Drawing.Icon GetLargeIcon(string file)
{
var shinfo = new SHFILEINFO();
var hImgLarge = SHGetFileInfo(file, 0, ref shinfo, (uint)Marshal.SizeOf(shinfo), FileIcon.SHGFI_ICON | FileIcon.SHGFI_LARGEICON);
return System.Drawing.Icon.FromHandle(shinfo.hIcon);
}
public static System.Drawing.Icon GetSmallIcon(string file)
{
var shinfo = new SHFILEINFO();
var hImgLarge = SHGetFileInfo(file, 0, ref shinfo, (uint)Marshal.SizeOf(shinfo), FileIcon.SHGFI_ICON | FileIcon.SHGFI_SMALLICON);
return System.Drawing.Icon.FromHandle(shinfo.hIcon);
}
}
22617284-c3d3-4a4f-8daf-00924d264f58|0|.0
There are some instances when you may need to lock the mouse cursor to the screen. For example rotating the camera where you would press down on the right mouse button then drag the mouse to rotate the camera. If you wish to hide the mouse cursor and lock it to the screen while dragging you can use Screen.lockCursor.
using UnityEngine;
public class lockCursorExample : MonoBehaviour
{
void DidLockCursor()
{
Debug.Log("Locking cursor");
}
void DidUnlockCursor()
{
Debug.Log("Unlocking cursor");
}
private bool wasLocked;
void Update()
{
if (!Screen.lockCursor && this.wasLocked)
{
this.wasLocked = false;
this.DidUnlockCursor();
}
else if (Screen.lockCursor && !this.wasLocked)
{
this.wasLocked = true;
this.DidLockCursor();
}
if (Input.GetMouseButtonDown(1))
{
Screen.lockCursor = true;
}
if (Input.GetMouseButtonUp(1))
{
Screen.lockCursor = false;
}
}
}
a7e86638-b1c2-4ff2-b227-051c5b24eea2|0|.0
Ever wish you could have a tool tip appear when hovering your mouse over a control in the inspector? Well look no further then Unity’s ToolTip attribute that you can apply to your MonoBehaviour’s fields!
using UnityEngine;
using System.Collections;
public class ExampleClass : MonoBehaviour {
[Tooltip("Health value between 0 and 100.")]
public int health = 0;
}
fd3a3647-41bd-49fb-a6bc-9db16083c967|0|.0
Sometimes it is handy to keep a game object alive for as long as your game is running. In order to prevent your object from being destroyed when loading a new scene use GameObject.DontDestroyOnLoad and your game object will persist between scene changes.
5e886bdf-1a5b-4c74-a17d-55b2909bdd13|0|.0
If you need to retrieve the default Arial font via code at runtime you can do so using the Resources.GetBuiltInResource method. var font = Resources.GetBuiltinResource(typeof(Font), "Arial.ttf") as Font;
2827331f-d105-46b8-8951-7bc25c21c5a3|0|.0
If you need to stylize your gui you can use the GUISettings class to change, the cursor color, flash speed, the selection color for text fields, as well as double click behavior. public class GuiSettingsExample : MonoBehaviour
{
public Color cursorColor;
public float flashSpeed;
public bool doubleClickSelectWord;
public Color selectionColor;
public bool tripleCLickLine;
private string text = "test string";
private Vector2 scroll;
public GUISkin skin;
public void OnGUI()
{
GUI.skin = this.skin;
var settings = GUI.skin.settings;
settings.cursorColor = this.cursorColor;
settings.cursorFlashSpeed = this.flashSpeed;
settings.doubleClickSelectsWord = this.doubleClickSelectWord;
settings.selectionColor = this.selectionColor;
settings.tripleClickSelectsLine = this.tripleCLickLine;
this.scroll = GUILayout.BeginScrollView(this.scroll, false, false, GUILayout.ExpandWidth(true), GUILayout.ExpandHeight(true));
this.text = GUILayout.TextArea(this.text);
GUILayout.EndScrollView();
}
}
4df28504-3065-44e6-9b2f-8e66a7292bbe|0|.0
|
|