Calendar
Mo | Tu | We | Th | Fr | Sa | Su |
---|
30 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | 29 | 30 | 31 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
Archive
- 2002
- 2003
- 2004
- 2005
- 2006
- 2007
- 2008
- 2009
- 2010
- 2011
- 2012
- 2013
- 2014
- 2015
- 2016
- 2019
- 2020
|
Website may be up and down over next few months. I'm currently doing a complete overhaul of everything.
Going back to simple individual .htm pages, new overall site theme, sanitizing and cleaning up html of all
pages and blog posts, attempting to implement a new tooling and publishing system etc etc.
// <copyright>
// Copyright (c) 2012 Codefarts
// All rights reserved.
// contact@codefarts.com
// http://www.codefarts.com
// </copyright>
namespace Codefarts.ObjectPooling
{
using System;
/// <summary>
/// Provides a generic object pooling manager.
/// </summary>
/// <typeparam name="T"></typeparam>
public class ObjectPoolManager<T> where T : class
{
/// <summary>
/// Holds a reference to a singleton instance.
/// </summary>
private static ObjectPoolManager<T> instance;
/// <summary>
/// Used to track the number of items that have been pushed to the pool.
/// </summary>
private int count;
/// <summary>
/// Holds the pooled item references.
/// </summary>
private T[] cachedItems = new T[10000];
/// <summary>
/// Gets or sets the creation callback.
/// </summary>
public Func<T> CreationCallback { get; set; }
/// <summary>
/// Gets the number of items in the pool.
/// </summary>
public int Count
{
get
{
return this.count;
}
}
/// <summary>
/// Pops a item from the pool.
/// </summary>
/// <returns>A pooled object reference.</returns>
/// <exception cref="System.NullReferenceException">'CreationCallback' property must be set if you try to pop a item and there are no items available.</exception>
public T Pop()
{
// lock here to prevent treading conflicts with array manipulation
lock (this.cachedItems)
{
// check if there are any pooled objects
if (this.count < 1)
{
// check if creation callback is null
if (this.CreationCallback == null)
{
throw new NullReferenceException("'CreationCallback' property must be set if you try to pop a item and there are no items available.");
}
// there are no available objects so create a new one.
return this.CreationCallback();
}
// reduce the count
this.count--;
// retrieve the item and return it
return this.cachedItems[this.count];
}
}
/// <summary>
/// Pushes the specified value.
/// </summary>
/// <param name="value">The value to push into the pool.</param>
public void Push(T value)
{
// lock here to prevent treading conflicts with array manipulation
lock (this.cachedItems)
{
// update the count
this.count++;
// if we need more room for storage increase the size of the cache array
if (this.count > this.cachedItems.Length)
{
Array.Resize(ref this.cachedItems, this.cachedItems.Length * 2);
}
// store the value
this.cachedItems[this.count - 1] = value;
}
}
/// <summary>
/// Gets the singleton instance of the class.
/// </summary>
public static ObjectPoolManager<T> Instance
{
get
{
return instance ?? (instance = new ObjectPoolManager<T>());
}
}
}
}
And some unit tests to go along with it.
// <copyright>
// Copyright (c) 2012 Codefarts
// All rights reserved.
// contact@codefarts.com
// http://www.codefarts.com
// </copyright>
namespace Codefarts.Tests.ObjectPooling
{
using System;
using Codefarts.ObjectPooling;
using Microsoft.VisualStudio.TestPlatform.UnitTestFramework;
[TestClass]
public class ObjectPoolingManagerTests
{
ObjectPoolManager<TestObject> manager;
[TestInitialize]
public void Setup()
{
this.manager = new ObjectPoolManager<TestObject>();
}
[TestCleanup]
public void Cleanup()
{
this.manager = null;
}
public class TestObject
{
public string stringValue;
public int intValue;
}
[TestMethod]
public void Pop_With_Empty_Pool_NoCallback()
{
try
{
var item = this.manager.Pop();
Assert.IsNotNull(item);
}
catch (Exception ex)
{
Assert.IsTrue(ex is NullReferenceException);
}
}
[TestMethod]
public void Pop_With_Empty_Pool_WithCallback()
{
try
{
this.manager.CreationCallback = this.Callback;
var item = this.manager.Pop();
Assert.IsNotNull(item);
Assert.AreEqual(0, item.intValue);
Assert.AreEqual(null, item.stringValue);
}
catch (Exception ex)
{
Assert.IsTrue(ex is NullReferenceException);
}
}
private TestObject Callback()
{
return new TestObject();
}
[TestMethod]
public void Push_Object()
{
try
{
Assert.AreEqual(0, this.manager.Count);
this.manager.Push(new TestObject());
Assert.AreEqual(1, this.manager.Count);
}
catch (Exception ex)
{
Assert.Fail(ex.ToString());
}
}
[TestMethod]
public void Push_Pop_Objects()
{
try
{
Assert.AreEqual(0, this.manager.Count);
for (var i = 0; i < 3; i++)
{
this.manager.Push(new TestObject() { stringValue = "Item" + i, intValue = i });
}
Assert.AreEqual(3, this.manager.Count);
for (var i = 3 - 1; i >= 0; i--)
{
var item = this.manager.Pop();
Assert.AreEqual(i, item.intValue);
Assert.AreEqual("Item" + i, item.stringValue);
}
Assert.AreEqual(0, this.manager.Count);
}
catch (Exception ex)
{
Assert.Fail(ex.ToString());
}
}
}
}
a6075769-d4e3-4634-9ad9-6bd31ae2249f|0|.0
Did you know that the GUI class has a matrix property that you can use to rotate and scale your gui elements.
The sample behavior that is provided below will scale and rotate a gui label in the center of the screen.
public class GuiMatrixDemo : MonoBehaviour
{
private float rotation;
/// <summary>
/// OnGUI is called for rendering and handling GUI events.
/// </summary>
public void OnGUI()
{
var matrix = GUI.matrix;
GUI.Label(new Rect(5, 5, 100, 20), "before matrix");
this.rotation += 15f * Time.deltaTime;
var scale = Mathf.Clamp((float)Math.Sin(Time.time) + 1 * 2, 1, 3);
GUI.matrix = Matrix4x4.TRS(new Vector3(Screen.width / 2, Screen.height / 2, 0), Quaternion.Euler(0, 0, this.rotation), Vector3.one * scale);
var size = GUI.skin.label.CalcSize(new GUIContent("test string"));
var rect = new Rect((-size.x / 2f), (-size.y / 2f), size.x, size.y);
GUI.Label(rect, "test string");
GUI.matrix = matrix;
GUI.Label(new Rect(5, 25, 100, 20), "after matrix");
}
}
2936e197-d8e8-4a41-b1e1-b28f52910f69|0|.0
Ever wish you could draw properties for serializable classes and script variables using your own gui logic? Unity 4 introduces the PropertyDrawer base class to do just that!
6b8a7716-227e-4887-91de-8f76804f96a8|0|.0
Source (adapted for GenericImage) -> http://stackoverflow.com/a/11176961/341706
/// <summary>
/// Rotates the image.
/// </summary>
/// <param name="image">
/// The source image to rotate.
/// </param>
/// <param name="angle">
/// The angle in degrees to rotate the image.
/// </param>
/// <param name="centerX">The x position of the rotation axis.</param>
/// <param name="centerY">The y position of the rotation axis.</param>
/// <returns>
/// Returns a new rotated image.
/// </returns>
public static GenericImage<T> Rotate<T>(this GenericImage<T> image, int centerX, int centerY, float angle)
{
// source (adapted for GenericImage) -> http://stackoverflow.com/a/11176961/341706
var radians = (Math.PI / 180) * angle;
var cos = Math.Cos(radians);
var sin = Math.Sin(radians);
var newImage = new GenericImage<T>(image.Width, image.Height);
for (var x = 0; x < image.Width; x++)
for (var y = 0; y < image.Height; y++)
{
var m = x - centerX;
var n = y - centerY;
var j = ((int)(m * cos + n * sin)) + centerX;
var k = ((int)(n * cos - m * sin)) + centerY;
if (j >= 0 && j < image.Width && k >= 0 && k < image.Height)
{
newImage[x, y] = image[j, k];
}
}
return newImage;
}
44f6028c-a763-4abd-b834-f4d1ed6833ce|0|.0
Not a unity specific tip but still a handy unity helper for creating a unity project from file explorer. *Works only for windows platforms.* Download the CreateUnityProject.reg file below or open up Notepad and paste the snippet below then save the file with a *.reg file extension. Next navigate to the file and right click on it and select “Merge” from the popup menu. You should now be able to create a new unity project by right clicking on a empty folder in File Explorer and selecting “Create unity project”.
CreateUnityProject.reg (488.00 bytes)
CreateUnityProject64.reg (476.00 bytes)
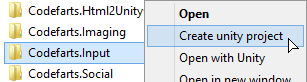
For x86 unity use the snippet below
Windows Registry Editor Version 5.00
[HKEY_CLASSES_ROOT\Folder\shell\Create unity project]
[HKEY_CLASSES_ROOT\Folder\shell\Create unity project\command]
@="\"C:\\Program Files (x86)\\Unity\\Editor\\unity.exe\" -createProject \"%1\""
For 64bit unity use the snippet below
Windows Registry Editor Version 5.00
[HKEY_CLASSES_ROOT\Folder\shell\Create unity project]
[HKEY_CLASSES_ROOT\Folder\shell\Create unity project\command]
@="\"C:\\Program Files\\Unity\\Editor\\unity.exe\" -createProject \"%1\""
55106668-07cb-46b3-a0b4-8535edc5800a|0|.0
Source -> http://stackoverflow.com/questions/10322341/simple-algorithm-for-drawing-filled-ellipse-in-c-c
/// <summary>
/// Draws a filled Ellipse.
/// </summary>
/// <param name="image">
/// The destination image.
/// </param>
/// <param name="x">
/// The x left most position of the ellipse.
/// </param>
/// <param name="y">
/// The y top most position of the ellipse.
/// </param>
/// <param name="width">
/// The width of the ellipse.
/// </param>
/// <param name="height">
/// The height of the ellipse.
/// </param>
/// <param name="color">
/// The color to use.
/// </param>
public static void FillEllipse<T>(this GenericImage<T> image, int x, int y, int width, int height, T color)
{
width = width / 2;
height = height / 2;
var centerX = x + width;
var centerY = y + height;
// source -> http://stackoverflow.com/questions/10322341/simple-algorithm-for-drawing-filled-ellipse-in-c-c
for (var indexY = -height; indexY <= height; indexY++)
{
for (var indexX = -width; indexX <= width; indexX++)
{
var dx = indexX / (double)width;
var dy = indexY / (double)height;
if (dx * dx + dy * dy <= 1)
{
image[centerX + indexX, centerY + indexY] = color;
}
}
}
}
fff06423-b3f9-4405-a901-8f0401b9141e|0|.0
Source (converted from C++) -> http://www.dailyfreecode.com/Code/draw-ellipse-midpoint-ellipse-algorithm-714.aspx
/// <summary>
/// Draws a Ellipse.
/// </summary>
/// <param name="image">
/// The destination image.
/// </param>
/// <param name="centerX">
/// The x center position of the circle.
/// </param>
/// <param name="centerY">
/// The y center position of the circle.
/// </param>
/// <param name="width">
/// The width of the Ellipse.
/// </param>
/// <param name="height">
/// The height of the Ellipse.
/// </param>
/// <param name="color">
/// The color to use.
/// </param>
public static void DrawEllipse<T>(this GenericImage<T> image, int centerX, int centerY, int width, int height, T color)
{
// source (converted from C++) -> http://www.dailyfreecode.com/Code/draw-ellipse-midpoint-ellipse-algorithm-714.aspx
float aa = (width * width);
float bb = (height * height);
float aa2 = (aa * 2);
float bb2 = (bb * 2);
float x = 0;
float y = height;
float fx = 0;
float fy = (aa2 * height);
float p = (int)(bb - (aa * height) + (0.25 * aa) + 0.5);
image[(int)(centerX + x), (int)(centerY + y)] = color;
image[(int)(centerX + x), (int)(centerY - y)] = color;
image[(int)(centerX - x), (int)(centerY - y)] = color;
image[(int)(centerX - x), (int)(centerY + y)] = color;
while (fx < fy)
{
x++;
fx += bb2;
if (p < 0) p += (fx + bb);
else
{
y--;
fy -= aa2;
p += (fx + bb - fy);
}
image[(int)(centerX + x), (int)(centerY + y)] = color;
image[(int)(centerX + x), (int)(centerY - y)] = color;
image[(int)(centerX - x), (int)(centerY - y)] = color;
image[(int)(centerX - x), (int)(centerY + y)] = color;
}
p = (int)((bb * (x + 0.5) * (x + 0.5)) + (aa * (y - 1) * (y - 1)) - (aa * bb) + 0.5);
while (y > 0)
{
y--;
fy -= aa2;
if (p >= 0) p += (aa - fy);
else
{
x++;
fx += bb2;
p += (fx + aa - fy);
}
image[(int)(centerX + x), (int)(centerY + y)] = color;
image[(int)(centerX + x), (int)(centerY - y)] = color;
image[(int)(centerX - x), (int)(centerY - y)] = color;
image[(int)(centerX - x), (int)(centerY + y)] = color;
}
}
19315d3b-b658-4a90-b895-b3a7ffec7a23|0|.0
Source -> http://www.dailyfreecode.com/code/fill-circle-scan-line-circle-fill-675.aspx
/// <summary>
/// Fills a circle.
/// </summary>
/// <param name="image">
/// The destination image.
/// </param>
/// <param name="centerX">
/// The x center position of the circle.
/// </param>
/// <param name="centerY">
/// The y center position of the circle.
/// </param>
/// <param name="radius">
/// The radius of the circle.
/// </param>
/// <param name="color">
/// The color to use.
/// </param>
public static void FillCircle<T>(this GenericImage<T> image, int centerX, int centerY, int radius, T color)
{
// source -> http://www.dailyfreecode.com/code/fill-circle-scan-line-circle-fill-675.aspx
int x1;
int x2;
var counter = (centerY + radius);
for (var count = (centerY - radius); count <= counter; count++)
{
x1 = (int)(centerX + Math.Sqrt((radius * radius) - ((count - centerY) * (count - centerY))) + 0.5);
x2 = (int)(centerX - Math.Sqrt((radius * radius) - ((count - centerY) * (count - centerY))) + 0.5);
image.DrawLine(x1, count, x2, count, color);
}
}
cd6a7ae2-c240-46ca-9a96-1a51b2ca790a|0|.0
I came across this article while searching for a way of unit testing my unity GUI windows. A link to the article is available here 50 Tips for Working with Unity (Best Practices). It contains some very sound advice and is well worth the time to read through.
31323acb-b1e0-4b40-bf75-17f7799067f1|1|1.0
Source (converted from Java) -> http://rosettacode.org/wiki/Bitmap/Midpoint_circle_algorithm#Java
/// <summary>
/// Draws a circle.
/// </summary>
/// <param name="image">
/// The destination image.
/// </param>
/// <param name="centerX">
/// The x center position of the circle.
/// </param>
/// <param name="centerY">
/// The y center position of the circle.
/// </param>
/// <param name="radius">
/// The radius of the circle.
/// </param>
/// <param name="color">
/// The color to use.
/// </param>
public static void DrawCircle<T>(this GenericImage<T> image, int centerX, int centerY, int radius, T color)
{
// source (converted from Java) -> http://rosettacode.org/wiki/Bitmap/Midpoint_circle_algorithm#Java
var d = (5 - radius * 4) / 4;
var x = 0;
var y = radius;
do
{
// ensure index is in range before setting (depends on your image implementation)
// in this case we check if the pixel location is within the bounds of the image before setting the pixel
image[centerX + x, centerY + y] = color;
image[centerX + x, centerY - y] = color;
image[centerX - x, centerY + y] = color;
image[centerX - x, centerY - y] = color;
image[centerX + y, centerY + x] = color;
image[centerX + y, centerY - x] = color;
image[centerX - y, centerY + x] = color;
image[centerX - y, centerY - x] = color;
if (d < 0)
{
d += 2 * x + 1;
}
else
{
d += 2 * (x - y) + 1;
y--;
}
x++;
} while (x <= y);
}
87e7dda7-bb65-4874-8846-50583b2109bd|0|.0
|
|